12 Python One-Liners to Boost Your Scikit-learn Skills
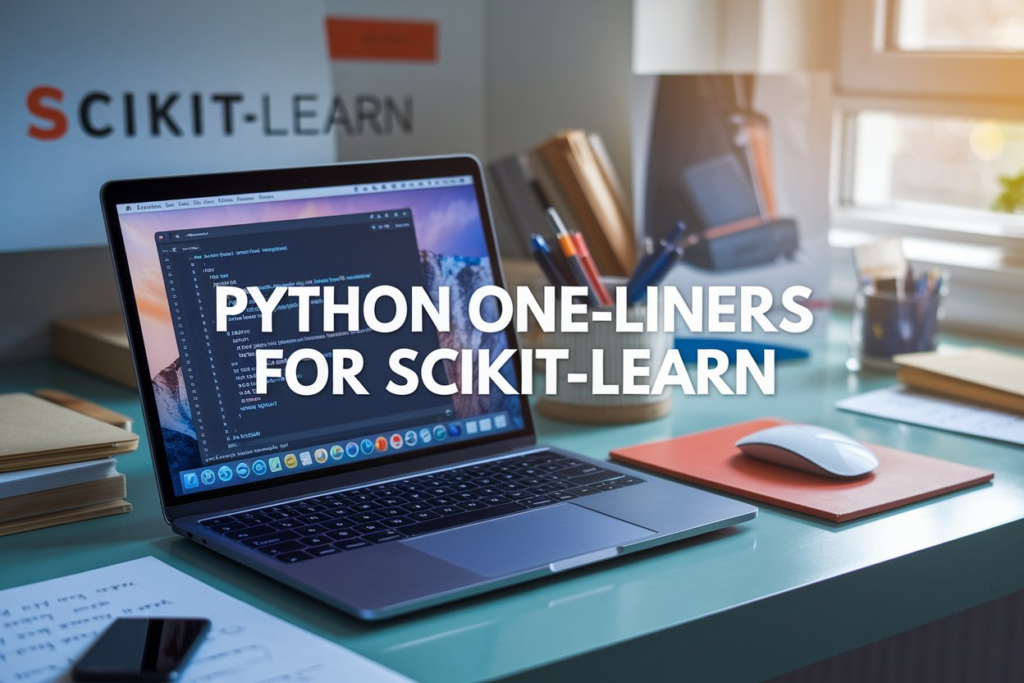
Introduction: 12 Python One-Liners to Boost Your Scikit-learn Skills
Python one-liners are short, powerful code snippets that can perform complex tasks in a single line. For Scikit-learn users, these concise expressions can streamline machine learning workflows, making code more efficient and easier to read.
Scikit-learn is a popular machine learning library for Python, offering a wide range of algorithms and tools for data preprocessing, model selection, and evaluation. By combining Scikit-learn's functionality with Python's expressive syntax, we can create one-liners that simplify common tasks in machine learning projects.
In this article, we'll explore 12 Python one-liners that can help you become more proficient with Scikit-learn. These compact code snippets will show you how to:
- Set up your environment quickly
- Load and prepare data efficiently
- Build and evaluate models with minimal code
- Perform advanced tasks like hyperparameter tuning and feature selection
By mastering these one-liners, you'll be able to write cleaner, more efficient code and focus more on the core aspects of your machine learning projects.
The Setup: Preparing Your Environment for Scikit-learn One-Liners
Before we dive into the one-liners, let's make sure your environment is set up correctly. You'll need Python installed on your system, along with the following libraries:
- Scikit-learn
- NumPy
- Pandas
- Matplotlib (for visualization)
To install these libraries, you can use pip, Python's package installer. Open your terminal or command prompt and run:
pip install scikit-learn numpy pandas matplotlib
Once the installation is complete, you can verify your setup by running Python and importing the libraries:
import sklearn
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
print(sklearn.__version__)
If you see the Scikit-learn version printed without any errors, you're ready to go!
One-Liner 1: Importing Essential Libraries in One Go
Our first one-liner sets the stage for your Scikit-learn projects by importing all the necessary libraries in a single line:
from sklearn import datasets, model_selection, metrics, preprocessing, ensemble; import numpy as np; import pandas as pd; import matplotlib.pyplot as plt
This line imports commonly used modules from Scikit-learn, as well as NumPy, Pandas, and Matplotlib. By using this one-liner at the start of your scripts or notebooks, you'll have quick access to a wide range of tools for data manipulation, model building, and visualization.
One-Liner 2: Loading and Splitting Data in a Single Step
Efficient data handling is crucial in machine learning workflows. This one-liner combines loading a dataset and splitting it into training and testing sets:
X, y = datasets.load_iris(return_X_y=True); X_train, X_test, y_train, y_test = model_selection.train_test_split(X, y, test_size=0.2, random_state=42)
This line loads the Iris dataset from Scikit-learn and immediately splits it into training and testing sets. The test_size=0.2
parameter reserves 20% of the data for testing, while random_state=42
ensures reproducibility.
You can easily adapt this one-liner for your own datasets:
data = pd.read_csv('your_dataset.csv'); X, y = data.drop('target', axis=1), data['target']; X_train, X_test, y_train, y_test = model_selection.train_test_split(X, y, test_size=0.2, random_state=42)
One-Liner 3: Creating Synthetic Datasets Quickly
Sometimes you need to generate synthetic data for testing or demonstration purposes. Scikit-learn provides functions to create datasets for both classification and regression tasks:
X, y = datasets.make_classification(n_samples=1000, n_features=20, n_classes=2, random_state=42)
This line creates a synthetic classification dataset with 1000 samples, 20 features, and 2 classes. You can adjust these parameters to suit your needs.
For regression tasks, you can use:
X, y = datasets.make_regression(n_samples=1000, n_features=10, noise=0.1, random_state=42)
These one-liners allow you to quickly generate datasets for experimenting with different algorithms or testing your code.
One-Liner 4: Building and Training Models with Minimal Code
Scikit-learn's consistent API allows us to initialize and train models in a single line:
model = ensemble.RandomForestClassifier(n_estimators=100, random_state=42).fit(X_train, y_train)
This line creates a Random Forest classifier with 100 trees and immediately trains it on the training data. You can easily swap out the model for other Scikit-learn estimators:
model = preprocessing.StandardScaler().fit_transform(X_train)
This one-liner scales the features of your training data using StandardScaler.
One-Liner 5: Evaluating Model Performance in a Snap
Once you've trained a model, you'll want to evaluate its performance. This one-liner predicts on the test set and calculates the accuracy score:
accuracy = metrics.accuracy_score(y_test, model.predict(X_test))
For a more robust evaluation using cross-validation, try this:
cv_scores = model_selection.cross_val_score(model, X, y, cv=5)
This performs 5-fold cross-validation and returns an array of scores.
One-Liner 6: Feature Scaling Simplified
Feature scaling is an important preprocessing step for many machine learning algorithms. This one-liner scales your features using StandardScaler:
X_scaled = preprocessing.StandardScaler().fit_transform(X)
This line fits the scaler to your data and transforms it in one go. You can easily switch to other scaling methods:
X_normalized = preprocessing.Normalizer().fit_transform(X)
One-Liner 7: Extracting Feature Importances Instantly
For tree-based models like Random Forests, you can quickly extract feature importances:
importances = ensemble.RandomForestClassifier(n_estimators=100, random_state=42).fit(X, y).feature_importances_
This line trains a Random Forest model and immediately retrieves the feature importance scores. You can use these scores to understand which features are most influential in your model's decisions.
One-Liner 8: Hyperparameter Tuning Made Compact
Hyperparameter tuning is crucial for optimizing model performance. This one-liner sets up and runs a grid search:
best_model = model_selection.GridSearchCV(ensemble.RandomForestClassifier(), {'n_estimators': [50, 100, 200], 'max_depth': [None, 10, 20]}, cv=5).fit(X, y).best_estimator_
This line performs a grid search over different combinations of n_estimators
and max_depth
for a Random Forest classifier, using 5-fold cross-validation. It then returns the best model found.
For a randomized search, which can be more efficient for large parameter spaces:
best_model = model_selection.RandomizedSearchCV(ensemble.RandomForestClassifier(), {'n_estimators': [50, 100, 200], 'max_depth': [None, 10, 20]}, n_iter=10, cv=5, random_state=42).fit(X, y).best_estimator_
One-Liner 9: Combining Pipelines with Model Training
Scikit-learn's Pipeline class allows you to chain multiple steps together. This one-liner creates a pipeline that scales the data and then trains a logistic regression model:
model = sklearn.pipeline.Pipeline([('scaler', preprocessing.StandardScaler()), ('clf', sklearn.linear_model.LogisticRegression())]).fit(X_train, y_train)
This approach ensures that the scaling is applied consistently to both training and test data, and makes it easy to apply the same preprocessing steps to new data when making predictions.
One-Liner 10: Visualizing Model Performance Without Boilerplate
Visualizing model performance can provide valuable insights. This one-liner creates a confusion matrix plot:
metrics.ConfusionMatrixDisplay.from_estimator(model, X_test, y_test).plot()
For ROC curve visualization:
metrics.RocCurveDisplay.from_estimator(model, X_test, y_test).plot()
These lines create and display the plots in one go, saving you from writing multiple lines of plotting code.
One-Liner 11: Exporting and Loading Models Efficiently
Saving and loading models is an important part of the machine learning workflow. This one-liner saves a trained model to a file:
from joblib import dump; dump(model, 'model.joblib')
And to load the model back:
from joblib import load; model = load('model.joblib')
These one-liners use joblib, which is generally faster than pickle for larger NumPy arrays.
One-Liner 12: Clustering or Dimensionality Reduction in a Flash
For unsupervised learning tasks, you can perform clustering or dimensionality reduction in one line:
clusters = sklearn.cluster.KMeans(n_clusters=3, random_state=42).fit_predict(X)
This line performs K-means clustering on your data, returning the cluster labels for each data point.
For dimensionality reduction using PCA:
X_reduced = sklearn.decomposition.PCA(n_components=2).fit_transform(X)
This reduces your data to two principal components, which can be useful for visualization or as a preprocessing step.
Tips for Writing Effective One-Liners in Scikit-learn
While one-liners can make your code more concise, it's important to use them judiciously. Here are some tips:
- Prioritize readability: If a one-liner becomes too complex, it might be better to break it into multiple lines.
- Use consistent naming conventions: Even in one-liners, use clear and consistent variable names.
- Comment your one-liners: A brief comment can help others (and your future self) understand what a complex one-liner does.
- Be mindful of performance: Some one-liners might be less efficient than their multi-line counterparts. Profile your code if performance is critical.
- Use one-liners for common patterns: If you find yourself repeating the same sequence of operations, consider creating a one-liner.
- Leverage method chaining: Many Scikit-learn methods return self, allowing you to chain multiple operations.
- Explore Scikit-learn's utility functions: Familiarize yourself with functions like
make_pipeline()
orcross_val_predict()
that can help create powerful one-liners. - Use list comprehensions and generator expressions: These Python features can help you create efficient one-liners for data transformation and filtering.
- Balance between one-liners and functions: For complex operations that you use frequently, consider creating a function instead of a long one-liner.
- Keep your environment consistent: One-liners often rely on having the right imports and variables in scope. Use consistent import statements and variable names across your project.
The Role of Python One-Liners in Machine Learning
Python one-liners, especially when used with Scikit-learn, can significantly streamline your machine learning workflows. They allow you to:
- Reduce boilerplate code: Common operations can be condensed into single lines, making your scripts cleaner and more focused on the core logic.
- Quickly prototype ideas: One-liners enable rapid experimentation with different models, preprocessing techniques, and evaluation methods.
- Improve code readability: Well-crafted one-liners can make your intentions clearer by encapsulating complete operations in a single expression.
- Enhance productivity: By reducing the amount of code you need to write for common tasks, one-liners can speed up your development process.
- Facilitate interactive exploration: In Jupyter notebooks or interactive Python sessions, one-liners allow you to quickly perform complex operations and see the results.
As you become more comfortable with Scikit-learn and Python's syntax, you'll likely find yourself naturally writing more one-liners. They'll become a valuable tool in your machine learning toolkit, allowing you to express complex ideas succinctly and efficiently.
Remember, the goal of using one-liners is not just to write shorter code, but to write more expressive and maintainable code. Use them where they enhance clarity and efficiency, but don't hesitate to break them up if they become too complex or hard to understand.
By mastering these 12 one-liners and understanding when and how to use them, you'll be well on your way to becoming more proficient with Scikit-learn and Python for machine learning. Happy coding!
More Articles for you
- WorkForceAi Review: This powerful AI platform empowers users to generate content, craft high-quality videos, produce AI voiceovers, automate tasks, and build AI chatbots and assistants
- Dubbify AI Review: An AI video platform that lets you paste any video URL to quickly localize, translate, and dub it into any desired language
- The Power of Gratitude: A Strategic Advantage in Business Success
- Ignite Your Business Growth: A Journey with Harrell Howard’s Game-Changing Playbook
- AI Teachify Review: Create Exceptional AI Courses 10 Times Faster with No Camera, No Course Creation, No Voice Recording, No Cost, and No Fancy Tools
- Femme Review: Create and monetize AI-powered supermodels. Say goodbye to expensive influencers and models.
- SmartLocal AI Review: Launch Your Automated SaaS in 3 Clicks to Build, Host, and Sell Websites to Local Businesses.