10 Python Scripts to Help You Automate Everyday Tasks
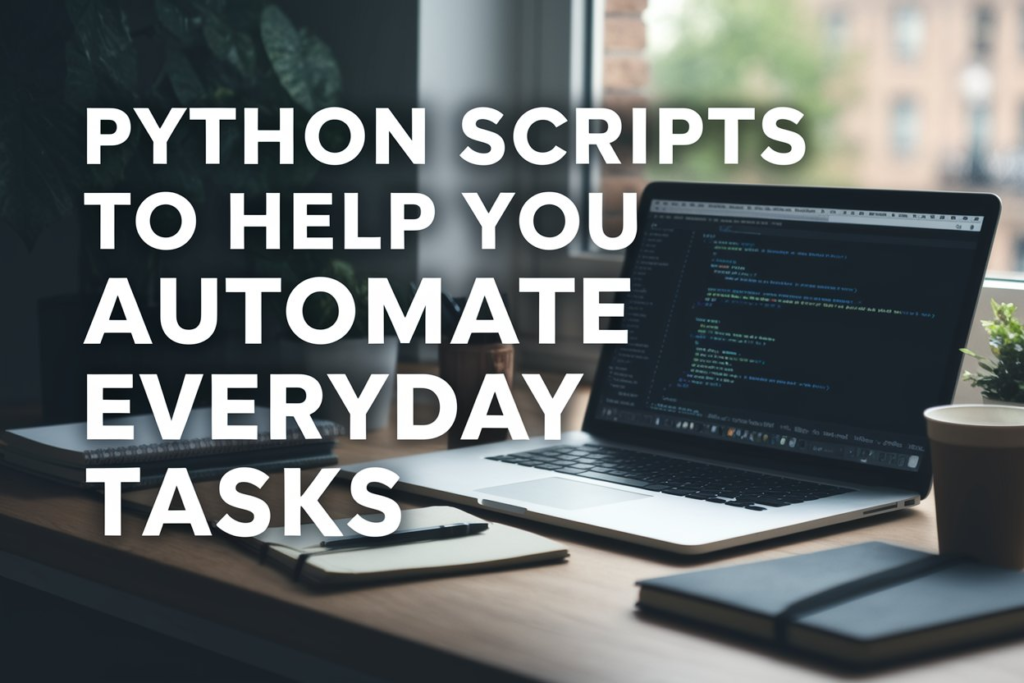
10 Python Scripts to Help You Automate Everyday Tasks
Unlocking the Potential of Python in Everyday Life
Hey there, fellow startup enthusiast! If you're anything like me, you're always on the lookout for ways to streamline your work and personal life. Well, let me introduce you to your new best friend: Python. This versatile programming language isn't just for hardcore developers – it's a secret weapon for anyone looking to automate those pesky, time-consuming tasks that eat away at our productivity.
Think about it. How many hours do you spend each week organizing emails, renaming files, or curating the perfect playlist? What if I told you that with just a few lines of code, you could automate all of that and more? That's the power of Python, and I'm here to show you how to harness it.
In this article, we'll dive into 10 practical Python scripts that can revolutionize your daily routine. Whether you're a coding newbie or a seasoned pro, these scripts are designed to be accessible, customizable, and, most importantly, incredibly useful. So, grab your favorite caffeinated beverage, and let's explore how Python can help you reclaim your time and sanity.
Script #1: Email Organizer for a Clutter-Free Inbox
The Problem: Inbox Overload
Let's kick things off with a universal headache: email management. We've all been there – you open your inbox on Monday morning, and it's a digital avalanche of unread messages, newsletters, and who-knows-what-else. It's enough to make you want to declare email bankruptcy and start over.
The Solution: Python to the Rescue
Enter our first Python script: the Email Organizer. This nifty piece of code uses the imaplib
and email
libraries to connect to your email account, scan your inbox, and automatically sort messages based on criteria you define. Think of it as your personal email assistant, working tirelessly behind the scenes to keep your inbox in check.
Here's a quick breakdown of what this script can do:
- Connect to your email server securely
- Scan incoming emails for specific keywords, senders, or dates
- Move matching emails to designated folders
- Archive old emails to keep your main inbox lean
The Code in Action
import imaplib
import email
import os
def connect_to_email(username, password):
imap_server = "imap.gmail.com"
imap_port = 993
mail = imaplib.IMAP4_SSL(imap_server, imap_port)
mail.login(username, password)
return mail
def organize_emails(mail):
mail.select('inbox')
# Search for emails from a specific sender
_, messages = mail.search(None, '(FROM "newsletter@example.com")')
for num in messages[0].split():
_, msg = mail.fetch(num, '(RFC822)')
email_body = msg[0][1]
email_message = email.message_from_bytes(email_body)
# Move to a 'Newsletters' folder
mail.copy(num, 'Newsletters')
mail.store(num, '+FLAGS', '\Deleted')
mail.expunge()
# Usage
username = "your_email@gmail.com"
password = "your_password"
mail = connect_to_email(username, password)
organize_emails(mail)
mail.logout()
This script is just scratching the surface. You can expand it to handle multiple rules, create new folders on the fly, or even integrate with your task management system to create to-dos from important emails.
The Time-Saving Magic
Imagine starting your day with a pre-sorted inbox. Important client emails are front and center, newsletters are neatly filed away for later reading, and ancient threads are archived. That's not just convenience – it's a superpower. You're saving time, reducing stress, and ensuring that nothing important slips through the cracks.
Script #2: Automated File Renaming Made Easy
The Problem: File Name Chaos
We've all been there – downloading a bunch of files only to find they have names like “IMG_20230415_123456.jpg” or “Document1.pdf”. It's a recipe for digital clutter and wasted time searching for specific files.
The Solution: Bulk Renaming with Python
Our second script tackles this head-on using Python's os
module and the powerful argparse
library for handling command-line arguments. This script can rename files in bulk based on rules you set, like adding date prefixes, sequential numbering, or content-based names.
The Code Breakdown
import os
import argparse
from datetime import datetime
def rename_files(directory, prefix, add_date):
for count, filename in enumerate(os.listdir(directory)):
if filename.endswith(('.jpg', '.jpeg', '.png', '.pdf')): # Add or remove file types as needed
new_name = f"{prefix}_{count+1}"
if add_date:
date_str = datetime.now().strftime("%Y%m%d")
new_name = f"{date_str}_{new_name}"
file_extension = os.path.splitext(filename)[1]
new_filename = f"{new_name}{file_extension}"
os.rename(os.path.join(directory, filename), os.path.join(directory, new_filename))
print(f"Renamed: {filename} to {new_filename}")
if __name__ == "__main__":
parser = argparse.ArgumentParser(description="Bulk rename files in a directory")
parser.add_argument("directory", help="Directory containing files to rename")
parser.add_argument("--prefix", default="File", help="Prefix for renamed files")
parser.add_argument("--add-date", action="store_true", help="Add date to filename")
args = parser.parse_args()
rename_files(args.directory, args.prefix, args.add_date)
Practical Applications
This script is a game-changer for scenarios like:
- Organizing photos from a vacation or event
- Standardizing document names for a project
- Preparing files for a client presentation
By automating this process, you're not just saving time – you're creating a system that makes file management a breeze.
Script #3: Daily To-Do List Generator
The Problem: Starting the Day Unfocused
How many times have you sat down at your desk, ready to tackle the day, only to realize you don't know where to start? Without a clear plan, it's easy to get lost in the weeds of busy work instead of focusing on what really matters.
The Solution: Automated To-Do List Creation
Our third script uses Python to generate a daily to-do list, pulling from a master list of tasks and prioritizing based on deadlines or categories. It's like having a personal assistant who knows exactly what you need to focus on each day.
The Code at Work
import csv
from datetime import datetime, timedelta
def generate_todo_list(master_list_file, output_file):
today = datetime.now().date()
tasks_for_today = []
with open(master_list_file, 'r') as file:
reader = csv.DictReader(file)
for row in reader:
task_date = datetime.strptime(row['due_date'], '%Y-%m-%d').date()
if task_date <= today + timedelta(days=1): # Include tasks due today or tomorrow
tasks_for_today.append(row)
# Sort tasks by priority
tasks_for_today.sort(key=lambda x: int(x['priority']))
with open(output_file, 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Task', 'Priority', 'Due Date'])
for task in tasks_for_today:
writer.writerow([task['task'], task['priority'], task['due_date']])
print(f"To-do list for {today} generated in {output_file}")
# Usage
generate_todo_list('master_tasks.csv', 'today_todo.csv')
This script reads from a CSV file containing all your tasks, their priorities, and due dates. It then creates a new CSV file with tasks due today or tomorrow, sorted by priority.
Integration and Expansion
To take this further, you could:
- Integrate with Google Calendar to add appointments to your daily list
- Use the
schedule
library to run this script automatically every morning - Create a simple GUI using
tkinter
to make the list interactive
The Mental Clarity Boost
Starting your day with a clear, prioritized list of tasks is like having a roadmap for productivity. It eliminates decision fatigue and ensures you're always working on what's most important. This isn't just about getting more done – it's about feeling in control of your day from the moment you start.
Script #4: Web Scraper for News Highlights
The Problem: Information Overload
In today's fast-paced world, staying informed is crucial. But with countless news sources and limited time, how do you keep up without spending hours scrolling through different websites?
The Solution: Personalized News Aggregator
Our fourth script uses Python's requests
library and BeautifulSoup
to scrape headlines and summaries from your favorite news sources, delivering a curated digest right to your inbox or a text file.
The Code in Action
import requests
from bs4 import BeautifulSoup
import csv
def scrape_news(url, selector):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
headlines = soup.select(selector)
return [headline.text.strip() for headline in headlines]
def save_headlines(headlines, filename):
with open(filename, 'w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerow(['Headline'])
for headline in headlines:
writer.writerow([headline])
# Example usage
tech_headlines = scrape_news('https://news.ycombinator.com', '.titlelink')
business_headlines = scrape_news('https://www.wsj.com', '.WSJTheme--headline--unZqjb45')
save_headlines(tech_headlines, 'tech_news.csv')
save_headlines(business_headlines, 'business_news.csv')
print("News headlines saved to CSV files.")
This script scrapes headlines from Hacker News and The Wall Street Journal, but you can easily modify it to include any news sources you prefer.
Ethical Considerations
When web scraping, it's crucial to:
- Check the website's robots.txt file for scraping guidelines
- Implement rate limiting to avoid overloading the server
- Use the data responsibly and respect copyright
Enriching Your Morning Routine
Imagine starting your day with a personalized news briefing, tailored to your interests and curated from trusted sources. This script doesn't just save time – it ensures you're always in the loop on the topics that matter most to you, whether that's tech trends, market updates, or industry-specific news.
Script #5: Expense Tracker That Writes Itself
The Problem: The Money Mystery
How many times have you looked at your bank statement and wondered, “Where did all my money go?” Keeping track of expenses is crucial for personal finance and business alike, but it's often a tedious, easily forgotten task.
The Solution: Automated Expense Logging
Our fifth script takes the pain out of expense tracking by automatically parsing bank transaction emails or CSV files to generate comprehensive expense reports. It's like having a personal accountant working 24/7.
The Code Breakdown
import pandas as pd
import matplotlib.pyplot as plt
import re
def parse_transaction_email(email_content):
# This is a simplified example. You'd need to adapt this to your bank's email format
amount = re.search(r'\$(\d+\.\d{2})', email_content).group(1)
category = re.search(r'Category: (\w+)', email_content).group(1)
return float(amount), category
def generate_expense_report(transactions_file):
df = pd.read_csv(transactions_file)
# Group by category and sum the amounts
category_totals = df.groupby('Category')['Amount'].sum()
# Create a pie chart
plt.figure(figsize=(10, 8))
plt.pie(category_totals, labels=category_totals.index, autopct='%1.1f%%')
plt.title('Expense Breakdown by Category')
plt.savefig('expense_breakdown.png')
print("Expense report generated and saved as 'expense_breakdown.png'")
# Example usage
# Assume we have a CSV file 'transactions.csv' with columns 'Date', 'Amount', 'Category'
generate_expense_report('transactions.csv')
This script reads transaction data from a CSV file, categorizes expenses, and generates a visual breakdown using matplotlib. You can expand this to include:
- Automatic email parsing using the
email
library - Integration with bank APIs for real-time data
- Monthly or weekly report generation scheduled with
cron
jobs
The Financial Clarity Advantage
By automating your expense tracking, you're not just saving time – you're gaining invaluable insights into your spending habits. This data becomes a powerful tool for budgeting, identifying areas for cost-cutting, and making informed financial decisions. For startups, this level of financial awareness can be the difference between burning through cash and achieving sustainable growth.
Script #6: Social Media Post Scheduler
The Problem: Inconsistent Social Media Presence
In the world of startups, maintaining a strong social media presence is crucial. But between product development, customer meetings, and a million other tasks, consistently posting updates can fall by the wayside.
The Solution: Automated Social Media Management
Our sixth script leverages Python to schedule and publish posts across platforms like Twitter and Instagram. By batching your content creation and letting the script handle posting, you ensure a consistent online presence without the daily hassle.
The Code in Action
import tweepy
import schedule
import time
from datetime import datetime, timedelta
# Twitter API credentials
consumer_key = "YOUR_CONSUMER_KEY"
consumer_secret = "YOUR_CONSUMER_SECRET"
access_token = "YOUR_ACCESS_TOKEN"
access_token_secret = "YOUR_ACCESS_TOKEN_SECRET"
# Authenticate with Twitter
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth)
def post_tweet(message):
try:
api.update_status(message)
print(f"Tweet posted: {message}")
except Exception as e:
print(f"Error posting tweet: {e}")
def schedule_tweets(tweets):
for i, tweet in enumerate(tweets):
schedule_time = datetime.now() + timedelta(days=i)
schedule.every().day.at(schedule_time.strftime("%H:%M")).do(post_tweet, tweet)
# Example usage
tweets_to_post = [
"Excited to announce our latest feature! #NewRelease #TechStartup",
"Join us for a live demo this Friday at 2 PM EST. RSVP now!",
"Customer spotlight: How Company X increased efficiency by 200% with our solution."
]
schedule_tweets(tweets_to_post)
# Keep the script running
while True:
schedule.run_pending()
time.sleep(60)
This script uses the tweepy
library to interact with Twitter's API and the schedule
library to manage posting times. You can expand this to:
- Support multiple social media platforms
- Pull content from a CSV file or database
- Implement analytics tracking to measure post performance
Setting Up API Access
To use this script, you'll need to:
- Create a developer account on the platform (e.g., Twitter Developer Portal)
- Create an app to get API keys and access tokens
- Securely store these credentials (consider using environment variables)
The Consistency Advantage
By automating your social media posts, you're not just saving time – you're creating a more professional and engaging online presence. This consistent activity keeps your audience engaged and can lead to increased visibility, more followers, and ultimately, more opportunities for your startup.
Script #7: Bulk Image Resizer and Compressor
The Problem: Image Overload
Whether you're preparing product photos for your e-commerce site or organizing screenshots for a pitch deck, dealing with images can be a major time sink. Resizing and compressing images one by one is tedious and inefficient.
The Solution: Python-Powered Image Processing
Our seventh script uses the Pillow library to resize and compress images in bulk. This not only saves time but ensures consistency across all your visual assets.
The Code Breakdown
from PIL import Image
import os
def resize_and_compress(input_folder, output_folder, size=(800, 600), quality=85):
if not os.path.exists(output_folder):
os.makedirs(output_folder)
for filename in os.listdir(input_folder):
if filename.endswith(('.png', '.jpg', '.jpeg')):
input_path = os.path.join(input_folder, filename)
output_path = os.path.join(output_folder, filename)
with Image.open(input_path) as img:
# Resize
img.thumbnail(size)
# Save with compression
img.save(output_path, optimize=True, quality=quality)
print(f"Processed: {filename}")
# Example usage
input_folder = "raw_images"
output_folder = "processed_images"
resize_and_compress(input_folder, output_folder)
This script takes images from an input folder, resizes them to a specified dimension while maintaining aspect ratio, and saves them to an output folder with compression applied.
Practical Applications
This script is incredibly useful for:
- Preparing images for web use to improve page load times
- Standardizing image sizes for product catalogs
- Reducing file sizes for email attachments or cloud storage
The Time and Space Saving Magic
By automating image processing, you're not just saving time – you're ensuring consistency and optimizing your digital assets. This can lead to faster website load times, reduced storage costs, and a more professional look across all your visual content.
Script #8: Automated Birthday Reminder System
The Problem: Forgotten Celebrations
In the hustle of startup life, it's easy to forget important dates – team birthdays, client anniversaries, or even personal milestones. These missed opportunities can impact relationships and team morale.
The Solution: Python-Powered Personal Assistant
Our eighth script creates an automated birthday reminder system that sends personalized emails or text messages for upcoming celebrations.
The Code in Action
import csv
from datetime import datetime, timedelta
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email(subject, body, to_email):
# Your email configuration
from_email = "your_email@example.com"
password = "your_email_password"
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(from_email, password)
text = msg.as_string()
server.sendmail(from_email, to_email, text)
server.quit()
def check_birthdays(birthdays_file):
today = datetime.now().date()
upcoming = today + timedelta(days=7) # Check for birthdays in the next week
with open(birthdays_file, 'r') as file:
reader = csv.DictReader(file)
for row in reader:
name = row['name']
birthday = datetime.strptime(row['birthday'], '%Y-%m-%d').date().replace(year=today.year)
if today <= birthday <= upcoming:
days_until = (birthday - today).days
subject = f"Upcoming Birthday Reminder: {name}"
body = f"Don't forget! {name}'s birthday is in {days_until} days on {birthday.strftime('%B %d')}."
send_email(subject, body, "your_email@example.com")
print(f"Reminder sent for {name}'s birthday")
# Example usage
check_birthdays('birthdays.csv')
This script reads from a CSV file containing names and birthdays, checks for upcoming celebrations, and sends email reminders. You can enhance it by:
- Integrating with a CRM system for client birthdays
- Using the Twilio API to send text message reminders
- Adding customizable message templates for different types of celebrations
Personalizing the Experience
To make your reminders more impactful:
- Include personal notes or inside jokes in the messages
- Attach gift ideas based on the person's interests
- Set up different reminder schedules for different relationships (e.g., immediate team vs. clients)
The Relationship-Building Power
This script isn't just about remembering dates – it's about nurturing relationships. In the startup world, where every connection matters, these small gestures can make a big difference in building loyalty, whether with team members, clients, or partners.
Script #9: Spotify Playlist Curator
The Problem: Playlist Paralysis
Creating the perfect playlist for different moods, workouts, or events can be time-consuming and often results in the same old songs on repeat.
The Solution: AI-Powered Music Curation
Our ninth script uses the Spotify API to generate playlists based on mood, tempo, or genre, taking the guesswork out of music selection.
The Code Breakdown
import spotipy
from spotipy.oauth2 import SpotifyOAuth
scope = "playlist-modify-public"
sp = spotipy.Spotify(auth_manager=SpotifyOAuth(scope=scope))
def create_playlist(name, description):
user_id = sp.me()['id']
playlist = sp.user_playlist_create(user_id, name, public=True, description=description)
return playlist['id']
def add_tracks_to_playlist(playlist_id, tracks):
sp.playlist_add_items(playlist_id, tracks)
def generate_playlist(seed_tracks, target_energy, target_danceability, limit=30):
recommendations = sp.recommendations(seed_tracks=seed_tracks,
target_energy=target_energy,
target_danceability=target_danceability,
limit=limit)
return [track['uri'] for track in recommendations['tracks']]
# Example usage
seed_tracks = ["spotify:track:4iV5W9uYEdYUVa79Axb7Rh", "spotify:track:1301WleyT98MSxVHPZCA6M"]
playlist_id = create_playlist("My Energetic Workout Mix", "High-energy tracks to keep you motivated")
tracks = generate_playlist(seed_tracks, target_energy=0.8, target_danceability=0.7)
add_tracks_to_playlist(playlist_id, tracks)
print(f"Playlist created: https://open.spotify.com/playlist/{playlist_id}")
This script uses the spotipy
library to interact with Spotify's API. It creates a new playlist, generates track recommendations based on seed tracks and target attributes, and adds these tracks to the playlist.
Expanding the Functionality
You could enhance this script to:
- Analyze your listening history to generate personalized recommendations
- Create mood-based playlists using Spotify's audio features API
- Schedule playlist updates for different times of day or days of the week
The Soundtrack to Productivity
Music can significantly impact mood and productivity. By automating playlist creation, you're not just saving time – you're curating the perfect soundtrack for different aspects of your work and life. Whether it's focus music for deep work sessions, upbeat tracks for team meetings, or relaxing tunes for winding down, having the right music at your fingertips can be a game-changer.
Script #10: Desktop Cleaner for Digital Minimalism
The Problem: Digital Clutter
A cluttered desktop isn't just visually overwhelming – it can significantly slow down your computer and your productivity. But manually organizing files is time-consuming and often gets pushed to the bottom of the to-do list.
The Solution: Automated Desktop Organization
Our final script uses Python to automatically sort and organize files on your desktop, creating a clean, efficient workspace without any manual effort.
The Code in Action
import os
import shutil
from datetime import datetime
def organize_desktop(desktop_path):
# Define categories and their corresponding file extensions
categories = {
'Images': ['.jpg', '.jpeg', '.png', '.gif'],
'Documents': ['.pdf', '.doc', '.docx', '.txt'],
'Spreadsheets': ['.xls', '.xlsx', '.csv'],
'Presentations': ['.ppt', '.pptx'],
'Archives': ['.zip', '.rar', '.7z'],
'Code': ['.py', '.js', '.html', '.css']
}
# Create folders for each category if they don't exist
for category in categories:
category_path = os.path.join(desktop_path, category)
if not os.path.exists(category_path):
os.makedirs(category_path)
# Organize files
for filename in os.listdir(desktop_path):
file_path = os.path.join(desktop_path, filename)
if os.path.isfile(file_path):
file_ext = os.path.splitext(filename)[1].lower()
# Find the appropriate category for the file
for category, extensions in categories.items():
if file_ext in extensions:
new_path = os.path.join(desktop_path, category, filename)
shutil.move(file_path, new_path)
print(f"Moved {filename} to {category}")
break
else:
# If no category found, move to 'Miscellaneous'
misc_path = os.path.join(desktop_path, 'Miscellaneous')
if not os.path.exists(misc_path):
os.makedirs(misc_path)
new_path = os.path.join(misc_path, filename)
shutil.move(file_path, new_path)
print(f"Moved {filename} to Miscellaneous")
# Example usage
desktop_path = os.path.expanduser("~/Desktop")
organize_desktop(desktop_path)
This script categorizes files based on their extensions and moves them into appropriate folders. You can customize the categories and file types to suit your needs.
Enhancing the Script
To take this further, you could:
- Add a scheduling feature to run the script automatically at set intervals
- Implement a “quarantine” folder for files you haven't accessed in a long time
- Create a log file to keep track of moved files for easy reference
The Power of a Clean Workspace
A tidy desktop isn't just about aesthetics – it's about mental clarity and efficiency. By automating this organization process, you're creating an environment that promotes focus and reduces the mental load of visual clutter. It's a small change that can have a big impact on your daily productivity and stress levels.
Conclusion: Start Small, Automate Big
We've covered a lot of ground with these 10 Python scripts, each tackling a different aspect of daily life that can be streamlined through automation. The beauty of these scripts is that they're not just time-savers – they're game-changers in how you approach your work and personal life.
Remember, the goal isn't to automate everything at once. Start with one script that addresses your most pressing pain point. As you get comfortable with the process and see the benefits, you can gradually expand your automation toolkit.
Here are some key takeaways:
- Identify Repetitive Tasks: Look for patterns in your daily routine. If you find yourself doing something over and over, it's probably a good candidate for automation.
- Start Simple: Don't be intimidated by complex scripts. Even a simple automation can save you hours over time.
- Customize and Iterate: These scripts are starting points. Tailor them to your specific needs and continuously refine them as your needs change.
- Share the Knowledge: If you've created a script that's been a game-changer for you, share it with your team. Automation is even more powerful when it benefits the whole organization.
- Stay Curious: The world of Python and automation is vast and ever-evolving. Keep learning, exploring new libraries, and challenging yourself to find new ways to optimize your workflow.
By embracing automation, you're not just saving time – you're freeing up mental space to focus on the big-picture thinking that drives innovation and growth. Whether you're a solo entrepreneur or leading a growing startup, these Python scripts can be the secret weapons that give you an edge in today's fast-paced business world.
So, what are you waiting for? Pick a script, fire up your Python environment, and take that first step towards a more automated, efficient, and empowered way of working. The future of your productivity starts now!
More Articles for you
- WorkForceAi Review: This powerful AI platform empowers users to generate content, craft high-quality videos, produce AI voiceovers, automate tasks, and build AI chatbots and assistants
- Dubbify AI Review: An AI video platform that lets you paste any video URL to quickly localize, translate, and dub it into any desired language
- The Power of Gratitude: A Strategic Advantage in Business Success
- Ignite Your Business Growth: A Journey with Harrell Howard’s Game-Changing Playbook
- AI Teachify Review: Create Exceptional AI Courses 10 Times Faster with No Camera, No Course Creation, No Voice Recording, No Cost, and No Fancy Tools
- Femme Review: Create and monetize AI-powered supermodels. Say goodbye to expensive influencers and models.
- SmartLocal AI Review: Launch Your Automated SaaS in 3 Clicks to Build, Host, and Sell Websites to Local Businesses.